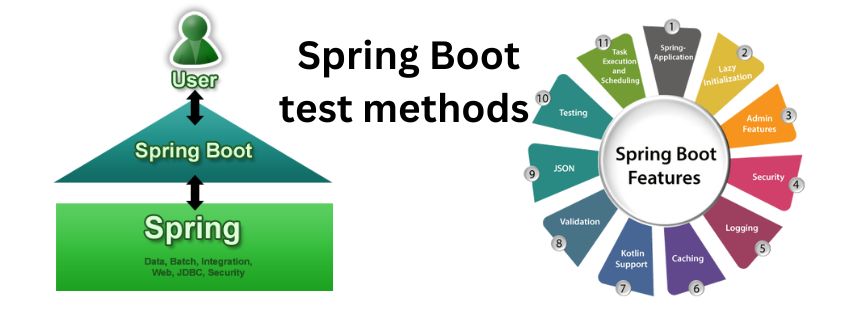
Jully 18, 2022
How to set header for Spring Boot test methods?
Setting a header for a Spring Boot test method is an important part of ensuring that the tests are properly configured and running correctly. This essay will discuss the various ways to set a header for a Spring Boot test method, including using the @Header annotation, setting the headers in the request, and using the MockMvcRequestBuilders. Additionally, this essay will discuss how to set multiple headers and how to set headers for specific requests. With this information, developers can ensure that their tests are properly configured and running correctly.
Using the @Header Annotation
The @Header annotation is a convenient way to set headers for a Spring Boot test method. This annotation takes two parameters: the header name and the header value. For example, if you want to set the “Content-Type” header to “application/JSON”, you can use the following code:
@Header(name = “Content-Type”, value = “application/JSON”)
This annotation can be used to set any header that is supported by Spring Boot. Additionally, this annotation can be used to set multiple headers in a single test method.
Setting the Headers in the Request
Another way to set headers for a Spring Boot test method is to set them in the request. This can be done by using the MockMvcRequestBuilders class. This class provides several methods for setting headers in a request, such as set header (), addHeader(), and add headers (). For example, if you want to set the “Content-Type” header to “application/JSON”, you can use the following code:
MockMvcRequestBuilders.set header(“Content-Type”, “application/JSON”)
This method can be used to set any header that is supported by Spring Boot. Additionally, this method can be used to set multiple headers in a single request.
Using the MockMvcRequestBuilders
The MockMvcRequestBuilders class also provides several methods for setting headers in a request. These methods include setHeader(), addHeader(), and addHeaders(). For example, if you want to set the “Content-Type” header to “application/JSON”, you can use the following code:
MockMvcRequestBuilders.set header(“Content-Type”, “application/JSON”)
This method can be used to set any header that is supported by Spring Boot. Additionally, this method can be used to set multiple headers in a single request.
Setting Multiple Headers
If you need to set multiple headers for a Spring Boot test method, you can use the MockMvcRequestBuilders class to do so. This class provides several methods for setting multiple headers in a request, such as add headers () and addHeader(). For example, if you want to set the “Content-Type” header to “application/JSON” and the “Accept” header to “application/XML”, you can use the following code:
MockMvcRequestBuilders.addHeaders(new Header(“Content-Type”, “application/json”), new Header(“Accept”, “application/xml”))
This method can be used to set any header that is supported by Spring Boot. Additionally, this method can be used to set multiple headers in a single request.
Setting Headers for Specific Requests
If you need to set headers for specific requests in a Spring Boot test method, you can use the MockMvcRequestBuilders class to do so. This class provides several methods for setting headers for specific requests, such as with header () and with headers (). For example, if you want to set the “Content-Type” header to “application/JSON” for a specific request, you can use the following code:
MockMvcRequestBuilders.with header(“Content-Type”, “application/JSON”)
This method can be used to set any header that is supported by Spring Boot. Additionally, this method can be used to set multiple headers in a single request.
In conclusion, setting a header for a Spring Boot test method is an important part of ensuring that the tests are properly configured and running correctly. There are several ways to do this, including using the @Header annotation, setting the headers in the request, and using the MockMvcRequestBuilders class. Additionally, developers can use these methods to set multiple headers and specific headers for specific requests. With this information, developers can ensure that their tests are properly configured and running correctly.
Recent Posts
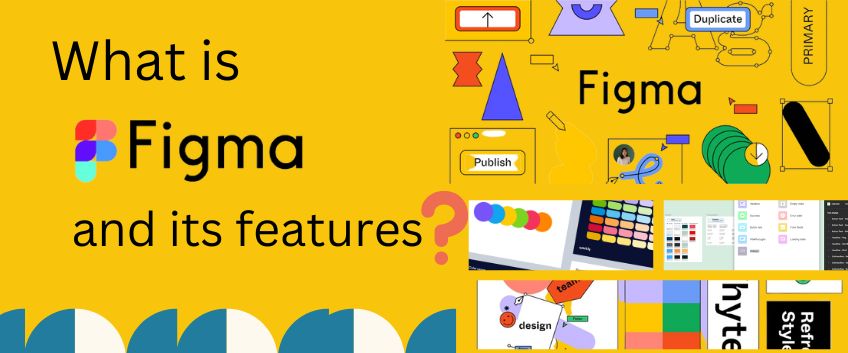
What is Figma and its features.
Jully 11, 2022
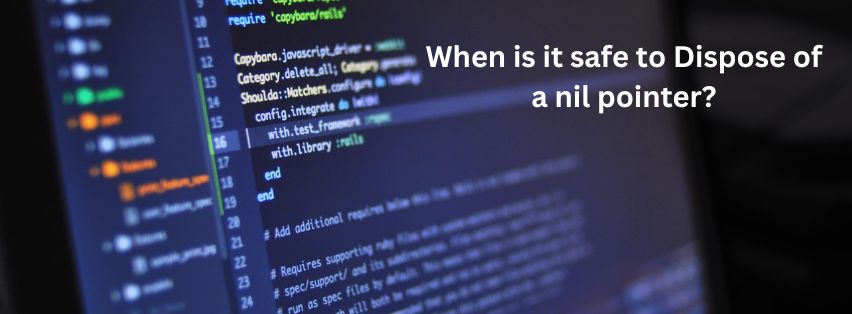
When is it safe to Dispose of a nil pointer?
Jully 11, 2022
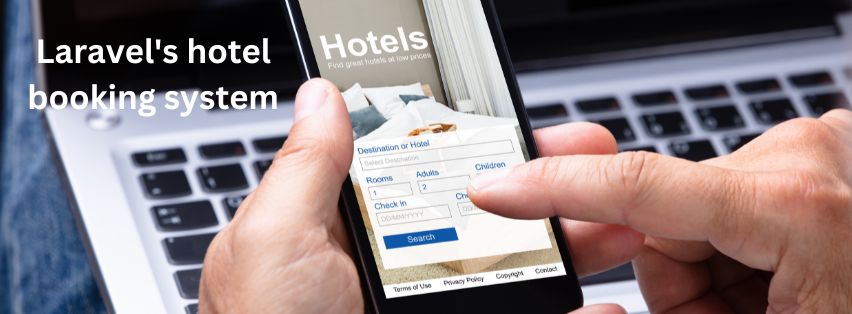
Introduction to Laravel’s hotel booking system.
Jully 11, 2022