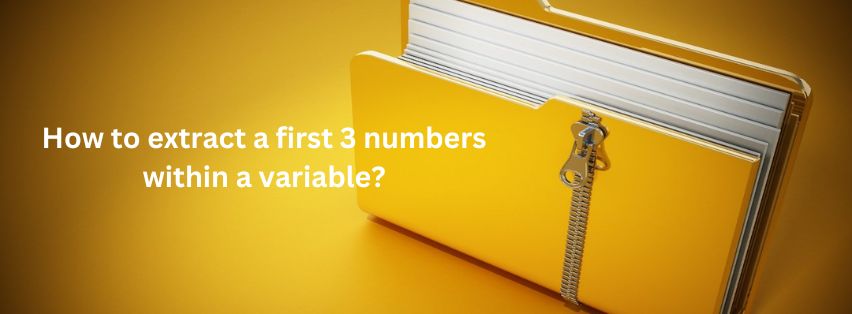
Jully 18, 2022
How to extract a first 3 numbers within a variable?
Extracting the first three numbers within a variable can be a useful tool for data analysis. It can be used to identify patterns, trends, and outliers in a dataset. This essay will discuss the various techniques for extracting the first three numbers within a variable, including using the substring function, regular expressions, and looping through the variable.
Using the Substring Function
The substring function is one of the most common methods for extracting the first three numbers within a variable. This function takes two parameters, the starting index and the length of the substring. For example, if we have a variable called “myVar” with the value “123456789”, we can use the substring function to extract the first three numbers by using the following code: myVar.substring(0,3). This will return the value “123”.
Using Regular Expressions
Regular expressions are another popular method for extracting the first three numbers within a variable. Regular expressions are powerful tools that allow you to search for patterns within strings. For example, if we have a variable called “myVar” with the value “123456789”, we can use the following regular expression to extract the first three numbers: /^\d{3}/. This will return the value “123”.
Looping Through the Variable
Looping through the variable is another technique for extracting the first three numbers within a variable. This method involves looping through each character in the variable and checking if it is a number. If it is a number, it is added to a new string until three numbers have been found. For example, if we have a variable called “myVar” with the value “123456789”, we can use the following code to extract the first three numbers:
String result = “”;
for (int i = 0; i < 3; i++) {
char c = myVar.charAt(i);
if (Character.isDigit(c)) {
result += c;
}
}
This will return the value “123”.
Using Arrays
Arrays are another useful tool for extracting the first three numbers within a variable. This method involves creating an array of characters from the variable and then looping through it to find the first three numbers. For example, if we have a variable called “myVar” with the value “123456789”, we can use the following code to extract the first three numbers:
char[] myArray = myVar.toCharArray();
String result = “”;
for (int i = 0; i < 3; i++) {
char c = myArray[i];
if (Character.isDigit(c)) {
result += c;
}
}
This will return the value “123”.
Using StringTokenizer
StringTokenizer is another useful tool for extracting the first three numbers within a variable. This method involves creating a StringTokenizer object from the variable and then looping through it to find the first three numbers. For example, if we have a variable called “myVar” with the value “123456789”, we can use the following code to extract the first three numbers:
StringTokenizer st = new StringTokenizer(myVar);
String result = “”;
while (st.hasMoreTokens()) {
String token = st.nextToken();
if (token.matches(“\\d+”)) {
result += token;
if (result.length() == 3) {
break;
}
This will return the value “123”.
Conclusion:
In conclusion, there are several techniques for extracting the first three numbers within a variable, including using the substring function, regular expressions, looping through the variable, using arrays, and using StringTokenizer. Each of these techniques has its own advantages and disadvantages, so it is important to choose the right one for your specific needs. With these techniques in mind, you should be able to easily extract the first three numbers within any variable.
Recent Posts
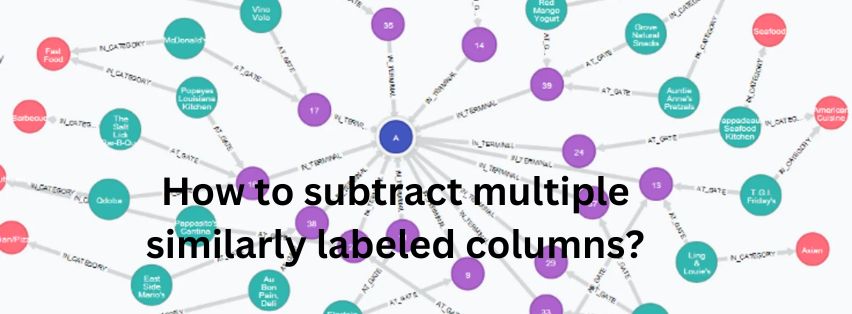
How to subtract multiple similarly labeled columns?
Jully 11, 2022
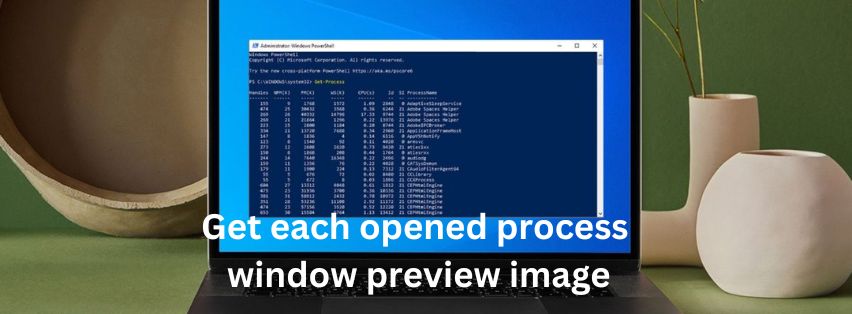
Get each opened process window preview image
Jully 11, 2022
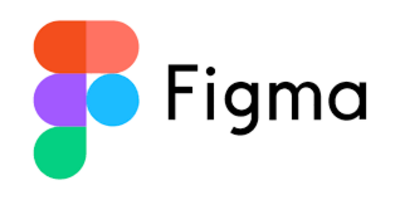
What is Figma and its features?
Jully 11, 2022