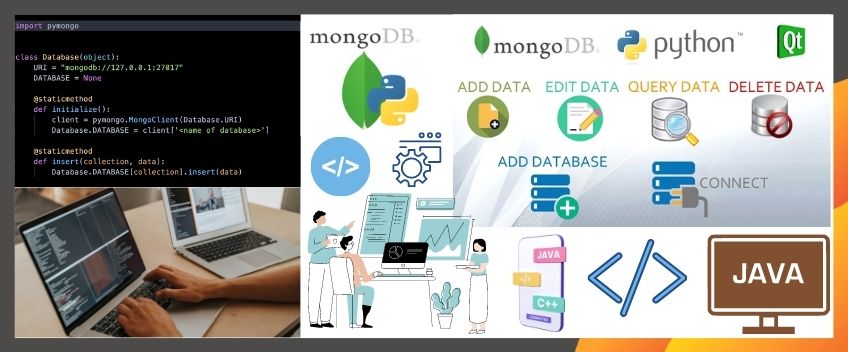
Jully 18, 2022
How to insert data in mongoDB using pymongo?
Data can be inserted into MongoDB using Mongo Shell, but we can also insert data into MongoDB using programming languages. In this article, we’ll discuss how to insert documents into MongoDB using the Python programming language.
Python
Python is a high-level programming language. This is one of the most popular and widely used programming languages. There are many reasons why developers love Python.
Its syntax is simple and easy to understand. It works perfectly with databases. It has an excellent collection of modules in its standard library.
Let us discuss How to insert data in MongoDB using pymongo.
Inserting data into MongoDB using Python
Before we begin, here is the fully working Python code. Go through it and then we will discuss the code step by step.
1 # importing pymongo
2 from pymongo import MongoClient 3 4 # establing connection 5 try: 6 connect = MongoClient() 7 print(“Connected successfully!!!”) 8 except: 9 print(“Could not connect to MongoDB”) 10 11 # connecting or switching to the database 12 db = connect.demoDB 13 14 # creating or switching to demoCollection 15 collection = db.demoCollection 16 17 # first document 18 document1 = { 19 “name”:”John”, 20 “age”:24, 21 “location”:”New York” 22 } 23 #second document 24 document2 = { 25 “name”:”Sam”, 26 “age”:21, 27 “location”:”Chicago” 28 } 29 30 # Inserting both document one by one 31 collection.insert_one(document1) 32 collection.insert_one(document2) 33 34 35 36 # Printing the data inserted 37 cursor = collection.find() 38 for record in cursor: 39 print(record) |
Let’s break this code down and understand exactly what’s going on.
Importing MongoClient and establishing the connection
Pymongo is used in Python to work with MongoDB. Since we’re inserting data into MongoDB, we first need to establish a connection to it. To establish the connection, we need MongoClient. In the first line of the program, we did exactly that.
from pymongo import MongoClient |
Now, we can use MongoClient in our programs. Let’s establish a connection.
connect = MongoClient() |
We used a variable – connection, to establish a connection with the mongo client. But here we can have exceptions like a connection to MongoClient failed. So in our program, we put the above in a try-catch block.
try:
connect = MongoClient() print(“Connected successfully!”) except: print(“Could not connect to MongoDB”) |
If the connection succeeds, the code is executed, otherwise, an exception is thrown.
Connecting to a database and to a collection
Now that we have a connection to MongoDB, we need to specify where the data should be inserted. Obviously, a database will exist. So to connect to a database, we’ll use the “connection” variable we created earlier when connecting to MongoClient.
db = connect.demoDB |
demo DB is a database. If the database is present, it will switch to it, otherwise, a new database will be created.
In a database, there is a collection. We need a collection now. We can use the “DB” variable to create a connection to a collection.
collection = db.demoCollection |
We now have a connection to the DemoDB database and DemoCollection collection.
Creating and Inserting Documents
The next step is to create the documents that will be inserted into demo collection. A MongoDB document should be produced in JSON format. Let’s build one.
document1 = {
“name”:”John”, “age”:24, “location”:”New York” } document2 = { “name”:”Sam”, “age”:21, “location”:”Chicago” } |
Each of the above documents has three fields – Name, Age, and Location.
It’s time for the important task – inserting data into the collection. To insert documents one after the other, we used the insert_one method.
collection.insert_one(document1)
collection.insert_one(document2) |
This way we insert data into MongoDB using Python.
Check the output
To verify, if the data was inserted correctly, we used the find method to retrieve all documents from the demo collection.
cursor = collection.find()
for record in cursor: print(record) |
Let’s examine the output.
Connected successfully!
{‘_id’: ObjectId(‘5d6bd4df25d75a89c4b1d4b0’), ‘name’: ‘John’, ‘age’: 24, ‘location’: ‘New York’} {‘_id’: ObjectId(‘5d6bd4df25d75a89c4b1d4b1’), ‘name’: ‘Sam’, ‘age’: 21, ‘location’: ‘Chicago’} |
Monitor the output. First, it says “Successfully connected!” And then it prints the document we inserted.
Finally
We can perform CRUD operations on MongoDB through Python programming language. It’s plain and simple. Here we used MongoClient to establish a connection and then insert data using the insert_one method. We hope you’ve learned what you need to solve your problem.
Recent Posts
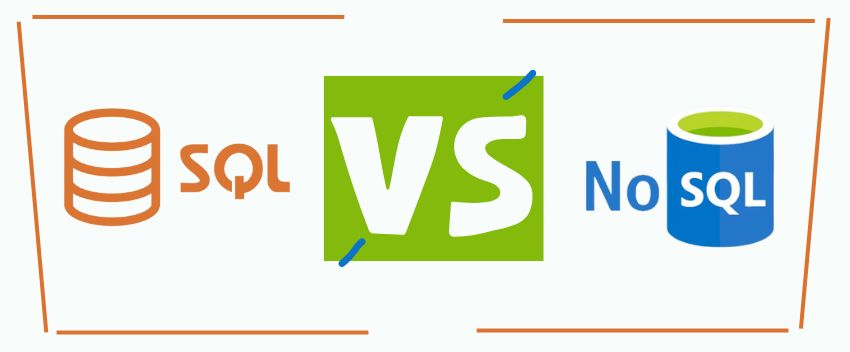
Difference between an SQL DB and a NoSQL DB
Jully 11, 2022
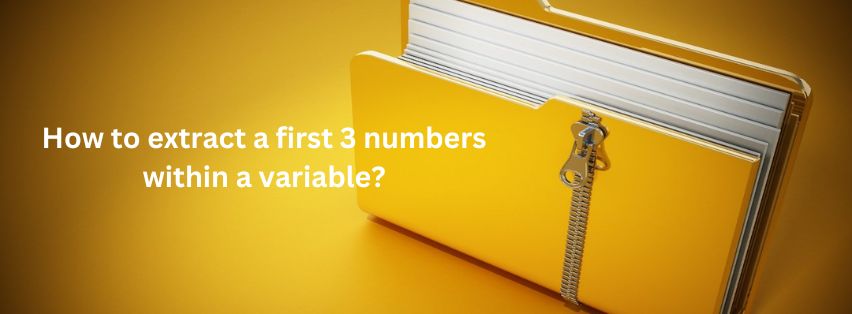
How to extract a first 3 numbers within a variable?
Jully 11, 2022
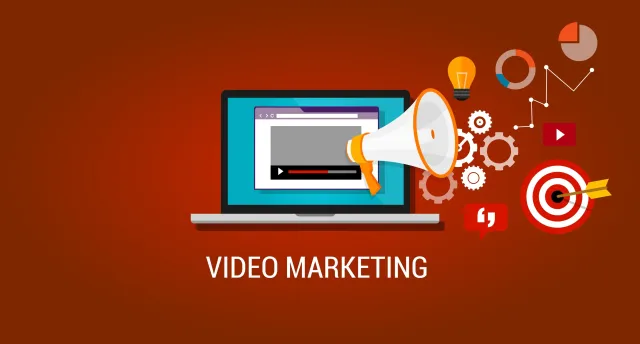
How to do video marketing on ecommerce store
Jully 11, 2022