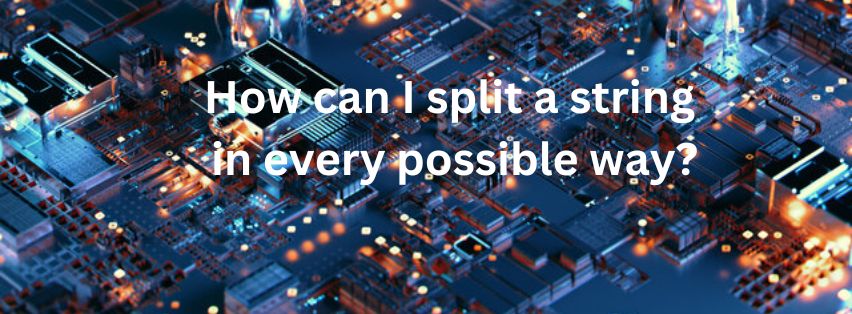
Jully 18, 2022
How can I split a string in every possible way?
Splitting a string is a common task in programming. It is a process of breaking down a string into smaller parts, or substrings, based on certain criteria. There are many ways to split a string, and each method has its own advantages and disadvantages. In this essay, I will discuss six different ways to split a string in every possible way, including using the split() method, using regular expressions, using the partition() method, using the replace() method, using the find() method, and using the looping technique.
Using the split() Method
The split() method is one of the most commonly used methods for splitting a string. This method takes a delimiter as an argument and splits the string based on that delimiter. For example, if we have a string “Hello World” and we want to split it on the space character, we can use the split() method like this: “Hello World”.split(” “). This will return an array containing two elements: “Hello” and “World”. The advantage of using this method is that it is very easy to use and understand. However, it can only be used to split a string based on a single delimiter.
Using Regular Expressions
Regular expressions are another way to split a string. Regular expressions are powerful tools that allow us to match patterns in strings. For example, if we have a string “Hello World” and we want to split it on any non-alphanumeric character, we can use a regular expression like this: “Hello World”.split(/\W+/). This will return an array containing two elements: “Hello” and “World”. The advantage of using regular expressions is that they are very powerful and can be used to match complex patterns in strings. However, they can be difficult to understand and use.
Using the partition() Method
The partition() method is another way to split a string. This method takes a delimiter as an argument and splits the string into three parts: the part before the delimiter, the delimiter itself, and the part after the delimiter. For example, if we have a string “Hello World” and we want to split it on the space character, we can use the partition() method like this: “Hello World”.partition(” “). This will return an array containing three elements: “Hello”, ” “, and “World”. The advantage of using this method is that it is very easy to use and understand. However, it can only be used to split a string based on a single delimiter.
Using the replace() Method
The replace() method is another way to split a string. This method takes two arguments: the substring to be replaced and the replacement substring. For example, if we have a string “Hello World” and we want to replace the space character with an underscore, we can use the replace() method like this: “Hello World”.replace(” “, “_”). This will return a new string with all spaces replaced by underscores: “Hello_World”. The advantage of using this method is that it is very easy to use and understand. However, it can only be used to replace one substring with another.
Using the find() Method
The find() method is another way to split a string. This method takes a substring as an argument and returns the index of the first occurrence of that substring in the string. For example, if we have a string “Hello World” and we want to find the index of the space character, we can use the find() method like this: “Hello World”.find(” “). This will return an integer value of 5, which is the index of the space character in the string. The advantage of using this method is that it is very easy to use and understand. However, it can only be used to find the index of a single substring in a string.
Using Looping Technique
The looping technique is another way to split a string. This technique involves looping through each character in the string and checking if it matches the criteria for splitting. For example, if we have a string “Hello World” and we want to split it on any non-alphanumeric character, we can use a loop like this:
let result = [];
let currentString = ”;
for (let i = 0; i < str.length; i++) {
const char = str[i];
if (/\W+/.test(char)) {
result.push(currentString);
currentString = ”;
} else {
currentString += char;
}
}
result.push(currentString);
This will return an array containing two elements: “Hello” and “World”. The advantage of using this technique is that it is very flexible and can be used to match any criteria for splitting. However, it can be difficult to understand and use.
Conclusion:
In conclusion, there are many ways to split a string in every possible way. The most common methods are using the split() method, using regular expressions, using the partition() method, using the replace() method, using the find() method, and using the looping technique. Each of these methods has its own advantages and disadvantages, so it is important to choose the right one for your specific needs. No matter which method you choose, you can be sure that you will be able to split your strings in every possible way.
Recent Posts
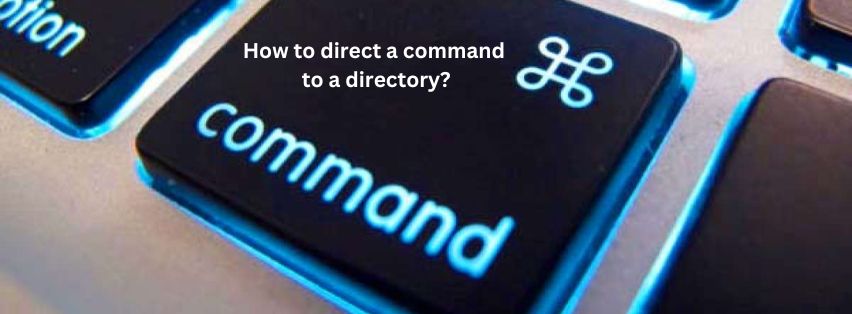
How to direct a command to a directory?
Jully 11, 2022
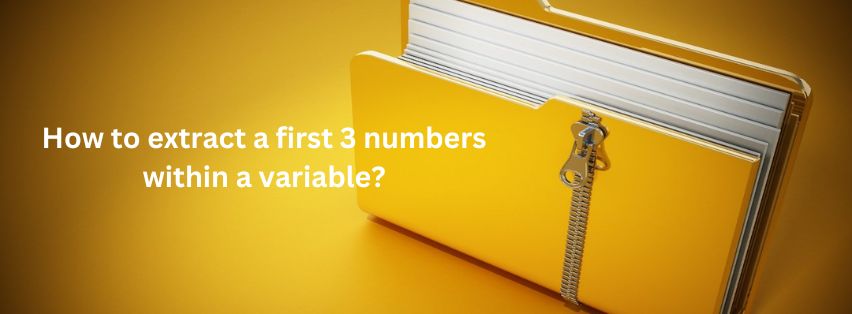
How to extract a first 3 numbers within a variable?
Jully 11, 2022
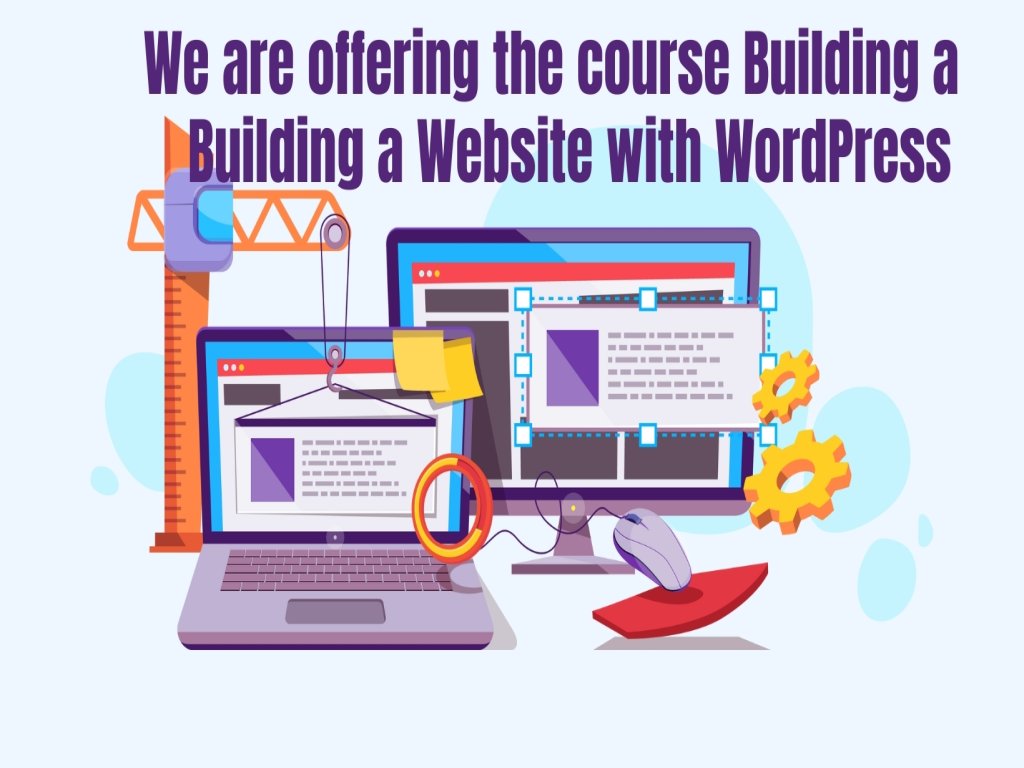
Build a Website with WordPress Course
Jully 11, 2022